
Google Sheets Integration
This MCP server integrates with your Google Drive and Google Sheets, to enable creating and modifying spreadsheets.
🤔 What is this?
mcp-google-sheets
is a Python-based MCP server that acts as a bridge between any MCP-compatible client (like Claude Desktop) and the Google Sheets API. It allows you to interact with your Google Spreadsheets using a defined set of tools, enabling powerful automation and data manipulation workflows driven by AI.
🚀 Quick Start (Using uvx
)
Essentially the server runs in one line: uvx mcp-google-sheets
.
This cmd will automatically download the latest code if needed and run it. However, it takes quite a few steps to setup the Google Cloud, please read the steps below.
-
☁️ Prerequisite: Google Cloud Setup
- You must configure Google Cloud Platform credentials and enable the necessary APIs first. We strongly recommend using a Service Account.
- ➡️ Jump to the Detailed Google Cloud Platform Setup guide below.
-
🐍 Install
uv
uvx
is part ofuv
, a fast Python package installer and resolver. Install it if you haven't already:
Follow instructions in the installer output to add# macOS / Linux curl -LsSf https://astral.sh/uv/install.sh | sh # Windows powershell -c "irm https://astral.sh/uv/install.ps1 | iex" # Or using pip: # pip install uv
uv
to your PATH if needed.
-
🔑 Set Essential Environment Variables (Service Account Recommended)
- You need to tell the server how to authenticate. Set these variables in your terminal:
- (Linux/macOS)
# Replace with YOUR actual path and folder ID from the Google Setup step export SERVICE_ACCOUNT_PATH="/path/to/your/service-account-key.json" export DRIVE_FOLDER_ID="YOUR_DRIVE_FOLDER_ID"
- (Windows CMD)
set SERVICE_ACCOUNT_PATH="C:\path\to\your\service-account-key.json" set DRIVE_FOLDER_ID="YOUR_DRIVE_FOLDER_ID"
- (Windows PowerShell)
$env:SERVICE_ACCOUNT_PATH = "C:\path\to\your\service-account-key.json" $env:DRIVE_FOLDER_ID = "YOUR_DRIVE_FOLDER_ID"
- ➡️ See Detailed Authentication & Environment Variables for other options (OAuth,
CREDENTIALS_CONFIG
).
-
🏃 Run the Server!
uvx
will automatically download and run the latest version ofmcp-google-sheets
:uvx mcp-google-sheets
- The server will start and print logs indicating it's ready.
-
🔌 Connect your MCP Client
- Configure your client (e.g., Claude Desktop) to connect to the running server.
- Depending on the client you use, you might not need step 4 because the client can launch the server for you. But it's a good practice to test run step 4 anyway to make sure things are set up properly.
- ➡️ See Usage with Claude Desktop for examples.
You're ready! Start issuing commands via your MCP client.
✨ Key Features
- Seamless Integration: Connects directly to Google Drive & Google Sheets APIs.
- Comprehensive Tools: Offers a wide range of operations (CRUD, listing, batching, sharing, formatting, etc.).
- Flexible Authentication: Supports Service Accounts (recommended), OAuth 2.0, and direct credential injection via environment variables.
- Easy Deployment: Run instantly with
uvx
(zero-install feel) or clone for development usinguv
. - AI-Ready: Designed for use with MCP-compatible clients, enabling natural language spreadsheet interaction.
🛠️ Available Tools & Resources
This server exposes the following tools for interacting with Google Sheets:
(Input parameters are typically strings unless otherwise specified)
list_spreadsheets
: Lists spreadsheets in the configured Drive folder (Service Account) or accessible by the user (OAuth).- Returns: List of objects
[{id: string, title: string}]
- Returns: List of objects
create_spreadsheet
: Creates a new spreadsheet.title
(string): The desired title.- Returns: Object with spreadsheet info, including
spreadsheetId
.
get_sheet_data
: Reads data from a range in a sheet.spreadsheet_id
(string)sheet
(string): Name of the sheet.range
(optional string): A1 notation (e.g.,'A1:C10'
,'Sheet1!B2:D'
). If omitted, reads the whole sheet.- Returns: 2D array of cell values.
update_cells
: Writes data to a specific range. Overwrites existing data.spreadsheet_id
(string)sheet
(string)range
(string): A1 notation.data
(2D array): Values to write.- Returns: Update result object.
batch_update_cells
: Updates multiple ranges in one API call.spreadsheet_id
(string)sheet
(string)ranges
(object): Dictionary mapping range strings (A1 notation) to 2D arrays of values{ "A1:B2": [[1, 2], [3, 4]], "D5": [["Hello"]] }
.- Returns: Batch update result object.
add_rows
: Appends rows to the end of a sheet (after the last row with data).spreadsheet_id
(string)sheet
(string)data
(2D array): Rows to append.- Returns: Update result object.
list_sheets
: Lists all sheet names within a spreadsheet.spreadsheet_id
(string)- Returns: List of sheet name strings
["Sheet1", "Sheet2"]
.
create_sheet
: Adds a new sheet (tab) to a spreadsheet.spreadsheet_id
(string)title
(string): Name for the new sheet.- Returns: New sheet properties object.
get_multiple_sheet_data
: Fetches data from multiple ranges across potentially different spreadsheets in one call.queries
(array of objects): Each object needsspreadsheet_id
,sheet
, andrange
.[{spreadsheet_id: 'abc', sheet: 'Sheet1', range: 'A1:B2'}, ...]
.- Returns: List of objects, each containing the query params and fetched
data
or anerror
.
get_multiple_spreadsheet_summary
: Gets titles, sheet names, headers, and first few rows for multiple spreadsheets.spreadsheet_ids
(array of strings)rows_to_fetch
(optional integer, default 5): How many rows (including header) to preview.- Returns: List of summary objects for each spreadsheet.
share_spreadsheet
: Shares a spreadsheet with specified users/emails and roles.spreadsheet_id
(string)recipients
(array of objects):[{email_address: '[email protected]', role: 'writer'}, ...]
. Roles:reader
,commenter
,writer
.send_notification
(optional boolean, default True): Send email notifications.- Returns: Dictionary with
successes
andfailures
lists.
add_columns
: Adds columns to a sheet. (Verify parameters if implemented)copy_sheet
: Duplicates a sheet within a spreadsheet. (Verify parameters if implemented)rename_sheet
: Renames an existing sheet. (Verify parameters if implemented)
MCP Resources:
spreadsheet://{spreadsheet_id}/info
: Get basic metadata about a Google Spreadsheet.- Returns: JSON string with spreadsheet information.
☁️ Google Cloud Platform Setup (Detailed)
This setup is required before running the server.
- Create/Select a GCP Project: Go to the Google Cloud Console.
- Enable APIs: Navigate to "APIs & Services" -> "Library". Search for and enable:
Google Sheets API
Google Drive API
- Configure Credentials: You need to choose one authentication method below (Service Account is recommended).
🔑 Authentication & Environment Variables (Detailed)
The server needs credentials to access Google APIs. Choose one method:
Method A: Service Account (Recommended for Servers/Automation) ✅
- Why? Headless (no browser needed), secure, ideal for server environments. Doesn't expire easily.
- Steps:
- Create Service Account: In GCP Console -> "IAM & Admin" -> "Service Accounts".
- Click "+ CREATE SERVICE ACCOUNT". Name it (e.g.,
mcp-sheets-service
). - Grant Roles: Add
Editor
role for broad access, or more granular roles (likeroles/drive.file
and specific Sheets roles) for stricter permissions. - Click "Done". Find the account, click Actions (⋮) -> "Manage keys".
- Click "ADD KEY" -> "Create new key" -> JSON -> "CREATE".
- Download and securely store the JSON key file.
- Click "+ CREATE SERVICE ACCOUNT". Name it (e.g.,
- Create & Share Google Drive Folder:
- In Google Drive, create a folder (e.g., "AI Managed Sheets").
- Note the Folder ID from the URL:
https://drive.google.com/drive/folders/THIS_IS_THE_FOLDER_ID
. - Right-click the folder -> "Share" -> "Share".
- Enter the Service Account's email (from the JSON file
client_email
). - Grant Editor access. Uncheck "Notify people". Click "Share".
- Set Environment Variables:
SERVICE_ACCOUNT_PATH
: Full path to the downloaded JSON key file.DRIVE_FOLDER_ID
: The ID of the shared Google Drive folder. (See Ultra Quick Start for OS-specific examples)
- Create Service Account: In GCP Console -> "IAM & Admin" -> "Service Accounts".
Method B: OAuth 2.0 (Interactive / Personal Use) 🧑💻
- Why? For personal use or local development where interactive browser login is okay.
- Steps:
- Configure OAuth Consent Screen: In GCP Console -> "APIs & Services" -> "OAuth consent screen". Select "External", fill required info, add scopes (
.../auth/spreadsheets
,.../auth/drive
), add test users if needed. - Create OAuth Client ID: In GCP Console -> "APIs & Services" -> "Credentials". "+ CREATE CREDENTIALS" -> "OAuth client ID" -> Type: Desktop app. Name it. "CREATE". Download JSON.
- Set Environment Variables:
CREDENTIALS_PATH
: Path to the downloaded OAuth credentials JSON file (default:credentials.json
).TOKEN_PATH
: Path to store the user's refresh token after first login (default:token.json
). Must be writable.
- Configure OAuth Consent Screen: In GCP Console -> "APIs & Services" -> "OAuth consent screen". Select "External", fill required info, add scopes (
Method C: Direct Credential Injection (Advanced) 🔒
- Why? Useful in environments like Docker, Kubernetes, or CI/CD where managing files is hard, but environment variables are easy/secure. Avoids file system access.
- How? Instead of providing a path to the credentials file, you provide the content of the file, encoded in Base64, directly in an environment variable.
- Steps:
- Get your credentials JSON file (either Service Account key or OAuth Client ID file). Let's call it
your_credentials.json
. - Generate the Base64 string:
- (Linux/macOS):
base64 -w 0 your_credentials.json
- (Windows PowerShell):
$filePath = "C:\path\to\your_credentials.json"; # Use actual path $bytes = [System.IO.File]::ReadAllBytes($filePath); $base64 = [System.Convert]::ToBase64String($bytes); $base64 # Copy this output
- (Caution): Avoid pasting sensitive credentials into untrusted online encoders.
- (Linux/macOS):
- Set the Environment Variable:
CREDENTIALS_CONFIG
: Set this variable to the full Base64 string you just generated.# Example (Linux/macOS) - Use the actual string generated export CREDENTIALS_CONFIG="ewogICJ0eXBlIjogInNlcnZpY2VfYWNjb..."
- Get your credentials JSON file (either Service Account key or OAuth Client ID file). Let's call it
Authentication Priority & Summary
The server checks for credentials in this order:
CREDENTIALS_CONFIG
(Base64 content)SERVICE_ACCOUNT_PATH
(Path to Service Account JSON)CREDENTIALS_PATH
(Path to OAuth JSON) - triggers interactive flow if token is missing/expired.
Environment Variable Summary:
Variable | Method(s) | Description | Default |
---|---|---|---|
SERVICE_ACCOUNT_PATH | Service Account | Path to the Service Account JSON key file. | - |
DRIVE_FOLDER_ID | Service Account | ID of the Google Drive folder shared with the Service Account. | - |
CREDENTIALS_PATH | OAuth 2.0 | Path to the OAuth 2.0 Client ID JSON file. | credentials.json |
TOKEN_PATH | OAuth 2.0 | Path to store the generated OAuth token. | token.json |
CREDENTIALS_CONFIG | Service Account / OAuth 2.0 | Base64 encoded JSON string of credentials content. | - |
⚙️ Running the Server (Detailed)
Method 1: Using uvx
(Recommended for Users)
As shown in the Ultra Quick Start, this is the easiest way. Set environment variables, then run:
uvx mcp-google-sheets
uvx
handles fetching and running the package temporarily.
Method 2: For Development (Cloning the Repo)
If you want to modify the code:
- Clone:
git clone https://github.com/yourusername/mcp-google-sheets.git && cd mcp-google-sheets
(Use actual URL) - Set Environment Variables: As described above.
- Run using
uv
: (Uses the local code)uv run mcp-google-sheets # Or via the script name if defined in pyproject.toml, e.g.: # uv run start
🔌 Usage with Claude Desktop
Add the server config to claude_desktop_config.json
under mcpServers
. Choose the block matching your setup:
🔵 Config: uvx + Service Account (Recommended)
{
"mcpServers": {
"google-sheets": {
"command": "uvx",
"args": ["mcp-google-sheets"],
"env": {
// Use ABSOLUTE paths here
"SERVICE_ACCOUNT_PATH": "/full/path/to/your/service-account-key.json",
"DRIVE_FOLDER_ID": "your_shared_folder_id_here"
},
"healthcheck_url": "http://localhost:8000/health" // Adjust host/port if needed
}
}
}
🔵 Config: uvx + OAuth 2.0
{
"mcpServers": {
"google-sheets": {
"command": "uvx",
"args": ["mcp-google-sheets"],
"env": {
// Use ABSOLUTE paths here
"CREDENTIALS_PATH": "/full/path/to/your/credentials.json",
"TOKEN_PATH": "/full/path/to/your/token.json" // Ensure this path is writable
},
"healthcheck_url": "http://localhost:8000/health"
}
}
}
(A browser may open for Google login on first use)
🔵 Config: uvx + CREDENTIALS_CONFIG (Service Account Example)
{
"mcpServers": {
"google-sheets": {
"command": "uvx",
"args": ["mcp-google-sheets"],
"env": {
// Paste the full Base64 string here
"CREDENTIALS_CONFIG": "ewogICJ0eXBlIjogInNlcnZpY2VfYWNjb3VudCIsCiAgInByb2plY3RfaWQiOiAi...",
"DRIVE_FOLDER_ID": "your_shared_folder_id_here" // Still needed for Service Account folder context
},
"healthcheck_url": "http://localhost:8000/health"
}
}
}
🟡 Config: Development (Running from cloned repo)
{
"mcpServers": {
"mcp-google-sheets-dev": { // Use a distinct name
"command": "uv",
"args": ["run", "mcp-google-sheets"], // Assumes `mcp-google-sheets` script exists
"cwd": "/full/path/to/cloned/mcp-google-sheets", // ABSOLUTE path to repo
"env": {
// Choose ONE auth method and set corresponding vars
// Example: Service Account Path
"SERVICE_ACCOUNT_PATH": "/full/path/to/cloned/mcp-google-sheets/service-account-key.json",
"DRIVE_FOLDER_ID": "your_shared_folder_id_here"
},
"healthcheck_url": "http://localhost:8000/health",
"disabled": false
}
}
}
💬 Example Prompts for Claude
Once connected, try prompts like:
- "List all spreadsheets I have access to." (or "in my AI Managed Sheets folder")
- "Create a new spreadsheet titled 'Quarterly Sales Report Q3 2024'."
- "In the 'Quarterly Sales Report' spreadsheet, get the data from Sheet1 range A1 to E10."
- "Add a new sheet named 'Summary' to the spreadsheet with ID
1aBcDeFgHiJkLmNoPqRsTuVwXyZ
." - "In my 'Project Tasks' spreadsheet, Sheet 'Tasks', update cell B2 to 'In Progress'."
- "Append these rows to the 'Log' sheet in spreadsheet
XYZ
:[['2024-07-31', 'Task A Completed'], ['2024-08-01', 'Task B Started']]
" - "Get a summary of the spreadsheets 'Sales Data' and 'Inventory Count'."
- "Share the 'Team Vacation Schedule' spreadsheet with
[email protected]
as a reader and[email protected]
as a writer. Don't send notifications."
🤝 Contributing
Contributions are welcome! Please open an issue to discuss bugs or feature requests. Pull requests are appreciated.
📄 License
This project is licensed under the MIT License - see the LICENSE file for details.
🙏 Credits
- Built with FastMCP.
- Inspired by kazz187/mcp-google-spreadsheet.
- Uses Google API Python Client libraries.
Stars
57Forks
10Last commit
5 days agoRepository age
1 monthLicense
MIT
Auto-fetched from GitHub .
MCP servers similar to Google Sheets Integration:
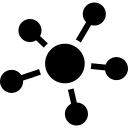
Stars
Forks
Last commit
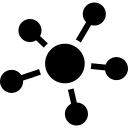
Stars
Forks
Last commit
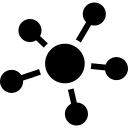
Stars
Forks
Last commit